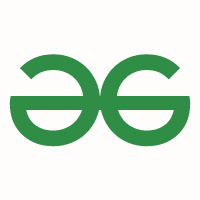
Sort a string without altering the position of vowels
Given a string S of size N, the task is to sort the string without changing the position of vowels.
Examples:
Input: S = “geeksforgeeks”
Output: feeggkokreess
Explanation:
The consonants present in the string are gksfrgks. Sorting the consonants modifies their sequence to fggkkrss.
Now, update the string by placing the sorted consonants in those positions.Input: S = “apple”
Output: alppe
Approach: Follow the steps below to solve the problem:
Below is the implementation of the above approach:
C++
|
feeggkokreess
Time Complexity: O(N logN)
Auxiliary Space: O(N)
Attention reader! Don’t stop learning now. Get hold of all the important DSA concepts with the DSA Self Paced Course at a student-friendly price and become industry ready.
0 comments:
Post a Comment