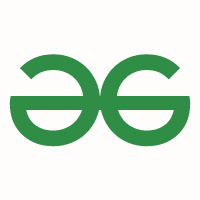
Kth element in permutation of first N natural numbers having all even numbers placed before odd numbers in increasing order
Given two integers N and K, the task is to find the Kth element in the permutation of first N natural numbers arranged such that all the even numbers appear before the odd numbers in increasing order.
Examples :
Input: N = 10, K = 3
Output: 6
Explanation:
The required permutation is {2, 4, 6, 8, 10, 1, 3, 5, 7, 9}.
The 3rd number in the permutation is 6.Input: N = 5, K = 4
Output: 3
Explanation:
The required permutation is {2, 4, 1, 3, 5}.
The 4th number in the permutation is 3.
Naive Approach: The simplest approach to solve the problem is to generate the required permutation of first N natural numbers and then traverse the permutation to find the Kth element present in it.
Follow the steps below to solve the problem:
- Initialize an array, say V[] of size N., to store the required sequence.
- Insert all even numbers less than or equal to N into V[].
- Then, insert all odd numbers less than or equal to N into V[].
- After forming the array, print the value of V[K – 1] as the result.
Below is the implementation of the above approach:
C++
|
Time Complexity: O(N)
Auxiliary Space: O(N)
Efficient Approach: To optimize the above approach, the idea is based on the observation that the first N / 2 elements are even and the value of the Kth element in the first half is equal to K * 2. If K > N/2, the value of the Kth element, depends on whether N is odd or even.
Follow the steps below to solve the problem:
- Initialize a variable, say ans, to store the Kth element.
- Check if the value of K ≤ N/2. If found to be true, update ans to K*2.
- Otherwise, K lies in the second half. In this case, ans depends on the value of N.
- Print the value of ans as the result.
Below is the implementation of the above approach:
C++
|
Time Complexity: O(1)
Auxiliary Space: O(1)
Attention reader! Don’t stop learning now. Get hold of all the important DSA concepts with the DSA Self Paced Course at a student-friendly price and become industry ready.
0 comments:
Post a Comment