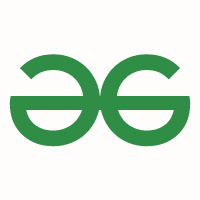
Modify array by sorting nearest perfect squares of array elements having their digits sorted in decreasing order
Given an array arr[] of size N (1 ≤ N ≤ 105), the task is to sort digits of each array element in descending order and replace each array element with the nearest perfect square and then print the array in ascending order.
Examples:
Input: arr[ ] = {29, 43, 28, 12}
Output: 25 49 81 100
Explanation:
Sorting digits of each array element in descending order modifies arr[] to {92, 43, 82, 21}.
Replacing elements with the nearest perfect square modifies arr[] to {100, 49, 81, 25}.
Sorting the array in ascending order modifies arr[] to {25, 49, 81, 100}.Input: arr[ ] = {517, 142, 905}
Output: 441 729 961
Approach: Follow the steps below to solve the problem:
Below is the implementation of the above approach.
C++
|
25 49 81 100
Time Complexity: O(NlogN)
Auxiliary Space: O(1)
Attention reader! Don’t stop learning now. Get hold of all the important DSA concepts with the DSA Self Paced Course at a student-friendly price and become industry ready.
0 comments:
Post a Comment