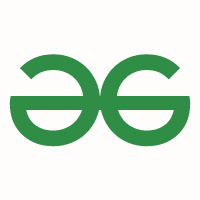
import
java.io.*;
public
class
Simple {
Node head =
null
;
Node tail =
null
;
class
Node {
int
data;
Node next;
Node(
int
d)
{
data = d;
next =
null
;
}
}
Node DeleteNodesfromBothEnds(
int
k)
{
if
(head ==
null
)
return
head;
Node firstPrev =
null
, secondPrev =
null
;
Node fast = head;
for
(
int
i =
0
; i < k -
1
; ++i) {
firstPrev = fast;
fast = fast.next;
}
Node slow = head;
while
(fast !=
null
&& fast.next !=
null
) {
secondPrev = slow;
slow = slow.next;
fast = fast.next;
}
if
(firstPrev == secondPrev) {
if
(firstPrev ==
null
) {
head = head.next;
}
else
{
firstPrev.next
= firstPrev.next.next;
}
}
else
if
(firstPrev !=
null
&& secondPrev !=
null
&& (firstPrev.next == secondPrev
|| secondPrev.next == firstPrev)) {
if
(firstPrev.next == secondPrev)
firstPrev.next
= secondPrev.next.next;
else
secondPrev.next
= firstPrev.next.next;
}
else
{
if
(firstPrev ==
null
) {
head = head.next;
}
else
{
firstPrev.next
= firstPrev.next.next;
}
if
(secondPrev ==
null
) {
head = head.next;
}
else
{
secondPrev.next
= secondPrev.next.next;
}
}
return
head;
}
public
void
push(
int
new_data)
{
Node new_node =
new
Node(new_data);
if
(head ==
null
) {
head = new_node;
tail = new_node;
}
else
{
tail.next = new_node;
tail = new_node;
}
}
public
void
printList()
{
Node tnode = head;
while
(tnode !=
null
) {
System.out.print(tnode.data +
" "
);
tnode = tnode.next;
}
}
public
static
void
main(String[] args)
{
Simple llist =
new
Simple();
llist.push(
1
);
llist.push(
2
);
llist.push(
3
);
llist.push(
4
);
llist.push(
5
);
llist.push(
6
);
int
K =
3
;
llist.DeleteNodesfromBothEnds(K);
llist.printList();
}
}
0 comments:
Post a Comment